Gatabase: Connection-Pooling Compile-time lightweight ORM for Postgres or SQLite.
- SQL DSL mimics SQL syntax!, API mimics stdlib!, Simple just 9 Templates!.
- Uses only system.nim, everything is done via template and macro, 0 Dependencies.
- Static Connection Pooling Array with 100+ ORM Queries.
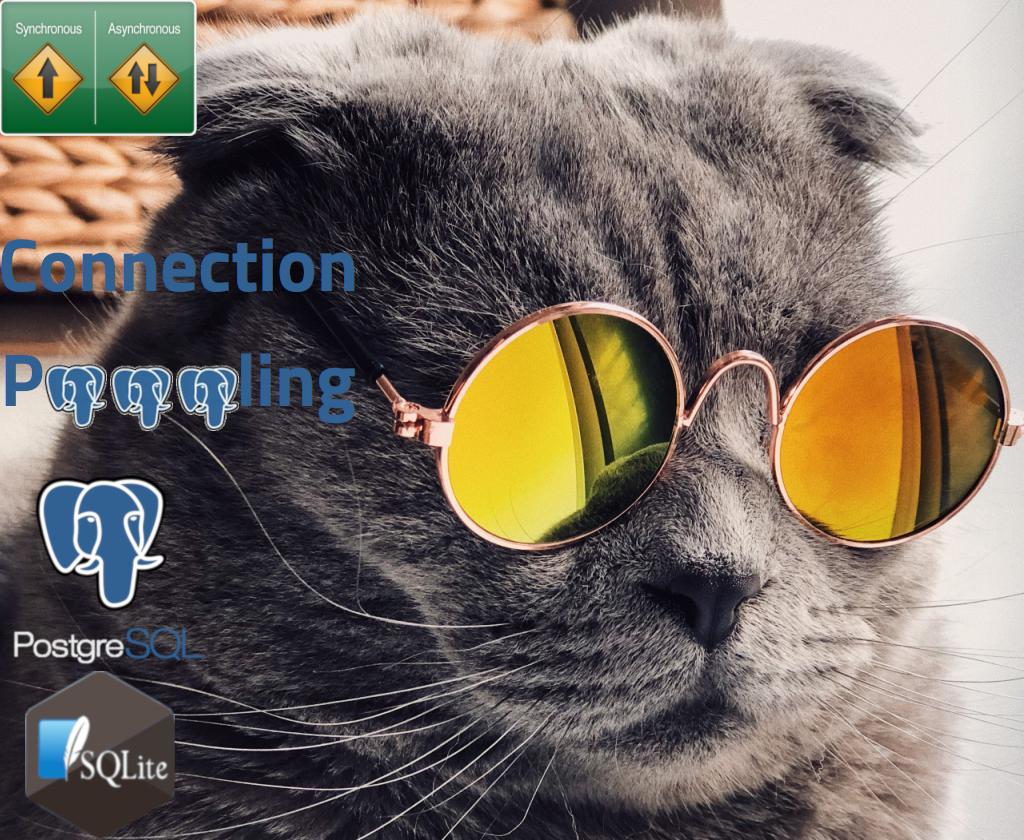
More Documentation
- Gatabase Sugar Recommended, but Optional, all Templates.
- DSL use https://github.com/juancarlospaco/nim-gatabase#gatabase
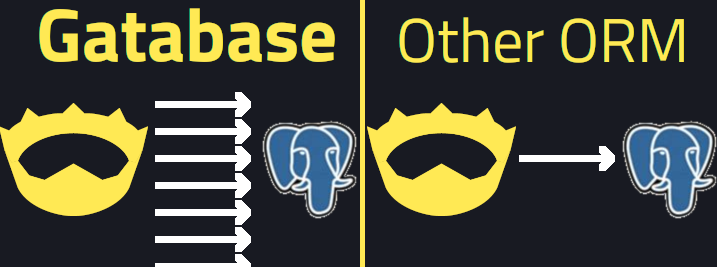
Templates
template exec(args: varargs[string, `$`] or seq[string]; inner: untyped)
-
Mimics exec but using Gatabase DSL.
- args are passed as-is to exec(), if no args use [], example [42, "OwO", true].
exec []: delete "person" where "active = false"
template tryExec(args: varargs[string, `$`] or seq[string]; inner: untyped): bool
-
Mimics tryExec but using Gatabase DSL.
- args are passed as-is to tryExec(), if no args use [], example [42, "OwO", true].
let killUser: bool = tryExec []: delete "person" where "id = 42"
let killUser: bool = tryExec []: select "name" `from` "person" wherenot "active = true"
template getRow(args: varargs[string, `$`] or seq[string]; inner: untyped): auto
-
Mimics getRow but using Gatabase DSL.
- args are passed as-is to getRow(), if no args use [], example [42, "OwO", true].
let topUser: Row = getAllRows []: selecttop "username" `from` "person" limit 1
template getAllRows(args: varargs[string, `$`] or seq[string]; inner: untyped): auto
-
Mimics getAllRows but using Gatabase DSL.
- args are passed as-is to getAllRows(), if no args use [], example [42, "OwO", true].
let allUsers: seq[Row] = [].getAllRows: select '*' `from` "person"
var allUsers: seq[Row] = getAllRows []: selectdistinct "names" `from` "person"
template getValue(args: varargs[string, `$`] or seq[string]; inner: untyped): string
-
Mimics getValue but using Gatabase DSL.
- args are passed as-is to getValue(), if no args use [], example [42, "OwO", true].
let userName: string = [].getValue: select "name" `from` "person" where "id = 42"
let age: string = getValue []: select "age" `from` "person" orderby DescNullsLast limit 1
template tryInsertID(args: varargs[string, `$`] or seq[string]; inner: untyped): int64
-
Mimics tryInsertID but using Gatabase DSL.
- args are passed as-is to tryInsertID(), if no args use [], example [42, "OwO", true].
let newUser: int64 = tryInsertID ["Graydon Hoare", "graydon.hoare@nim-lang.org"]: insertinto "person" values 2
template insertID(args: varargs[string, `$`] or seq[string]; inner: untyped): int64
-
Mimics insertID but using Gatabase DSL.
- args are passed as-is to insertID(), if no args use [], example [42, "OwO", true].
let newUser: int64 = ["Ryan Dahl", "ryan.dahl@nim-lang.org"].insertID: insertinto "person" values 2
template tryInsert(pkName: string; args: varargs[string, `$`] or seq[string]; inner: untyped): int64
-
Mimics tryInsert but using Gatabase DSL.
- args are passed as-is to tryInsert(), if no args use [], example [42, "OwO", true].
template insert(pkName: string; args: varargs[string, `$`] or seq[string]; inner: untyped): int64
-
Mimics insert but using Gatabase DSL.
- args are passed as-is to insertID(), if no args use [], example [42, "OwO", true].
template execAffectedRows(args: varargs[string, `$`] or seq[string]; inner: untyped): auto
-
Mimics execAffectedRows but using Gatabase DSL.
- args are passed as-is to execAffectedRows(), if no args use [], example [42, "OwO", true].
let activeUsers: int64 = execAffectedRows []: select "status" `from` "users" `--` "This is a SQL comment" where "status = true" isnull false
let distinctNames: int64 = execAffectedRows []: selectdistinct "name" `from` "users"
template getValue(args: varargs[string, `$`] or seq[string]; parseProc: proc; inner: untyped): auto
-
Alias for parseProc(getValue(db, sql("..."), args)). Returns actual value instead of string.
- parseProc is whatever proc parses the value of getValue(), any proc should work.
- args are passed as-is to getValue(), if no args use [], example [42, "OwO", true].
let age: int = getValue([], parseInt): select "age" `from` "users" limit 1
let ranking: float = getValue([], parseFloat): select "ranking" `from` "users" where "id = 42"
let preferredColor: string = [].getValue(parseHexStr): select "color" `from` "users" limit 1
template sqls(inner: untyped): auto
-
Build a SqlQuery using Gatabase ORM DSL, returns a vanilla SqlQuery.
const data: SqlQuery = sqls: select '*' `from` "users"
let data: SqlQuery = sqls: select "name" `from` "users" limit 9
var data: SqlQuery = sqls: delete '*' `from` "users"