Cloudbet Virtual Crypto Casino API Client
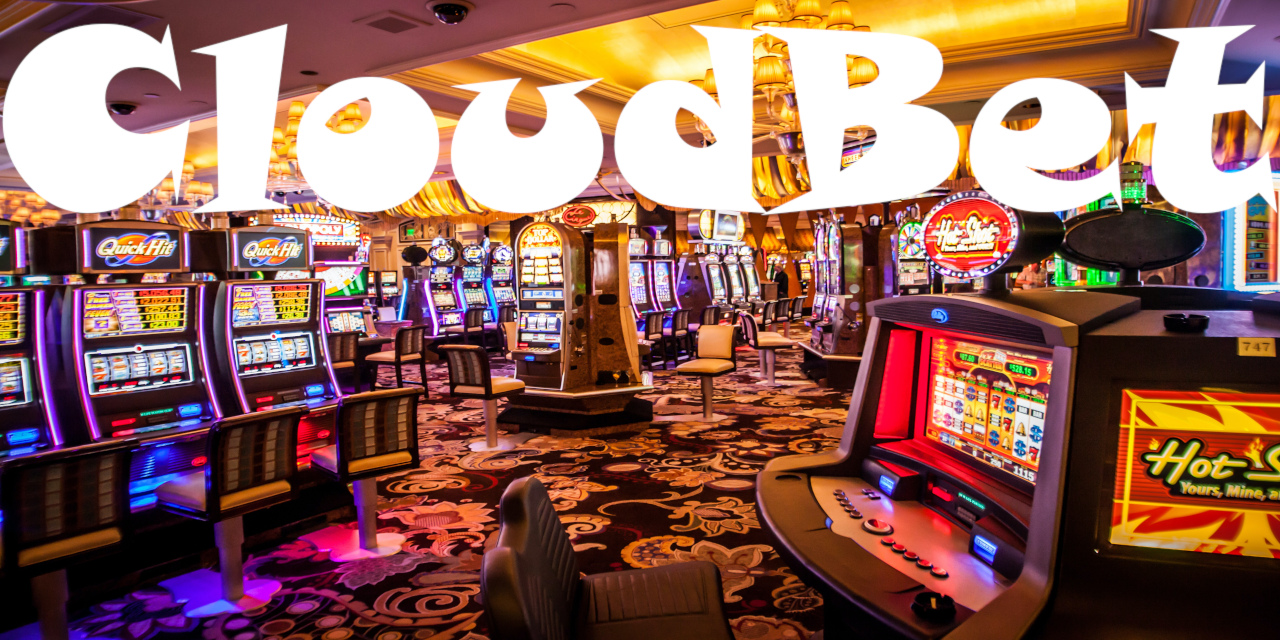
Example:
import src/cloudbet import std/[httpcore, uri] let client: Cloudbet = newCloudbet(apiKey = "YOUR_CLOUDBET_API_KEY") let preparedRequest = client.getSports() doAssert preparedRequest is tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string]
Types
AcceptPriceChange {.pure.} = enum NONE = "NONE", ALL = "ALL", BETTER = "BETTER"
- Accept price change.
Cloudbet = object apiKey*: string ## API Key for the Cloudbet API.
- Cloudbet client object.
CloudbetCurrency {.pure.} = enum BCH = "BCH", BTC = "BTC", CAD = "CAD", DAI = "DAI", DASH = "DASH", DOGE = "DOGE", ETH = "ETH", EUR = "EUR", LINK = "LINK", LTC = "LTC", PAX = "PAX", PAXG = "PAXG", PLAY_EUR = "PLAY_EUR", BONUS_EUR = "BONUS_EUR", USD = "USD", USDC = "USDC", USDT = "USDT"
- Currencies supported by Cloudbet.
CloudbetSport {.pure.} = enum american_football = "American Football", archery = "Archery", athletics = "Athletics", aussie_rules = "Aussie Rules", badminton = "Badminton", bandy = "Bandy", baseball = "Baseball", basketball = "Basketball", beach_soccer = "Beach Soccer", beach_volleyball = "Beach Volleyball", bowls = "Bowls", boxing = "Boxing", call_of_duty = "Call of Duty", chess = "Chess", counter_strike = "Counter-Strike", cricket = "Cricket", crossfire = "Crossfire", curling = "Curling", cycling = "Cycling", darts = "Darts", dota_2 = "Dota 2", entertainment = "Entertainment", esport_aoe = "Age of Empires", esport_arena_of_valor = "Arena of Valor", esport_bga = "BGA", esport_brawl_stars = "Brawl Stars", esport_fifa = "FIFA", esport_free_fire = "Free Fire", esport_king_of_glory = "King of Glory", esport_madden = "Madden", esport_nba2k = "NBA2K", esport_valorant = "Valorant", esport_warcraft = "Warcraft", field_hockey = "Field Hockey", floorball = "Floorball", formula_1 = "Formula 1", formula_e = "Formula E", futsal = "Futsal", golf = "Golf", greyhound = "Greyhound", handball = "Handball", hearthstone = "Hearthstone", heroes_of_the_storm = "Heroes of the Storm", horse_racing = "Horse Racing", ice_hockey = "Ice Hockey", kabaddi = "Kabaddi", league_of_legends = "League of Legends", mma = "MMA", motorsport = "Motorsport", olympics = "Olympics", overwatch = "Overwatch", pesapallo = "Pesapallo", politics = "Politics", rainbow_six = "Rainbow Six", rocket_league = "Rocket League", rugby_league = "Rugby League", rugby_union = "Rugby Union", sailing = "Sailing", snooker = "Snooker", soccer = "Soccer", specials = "Specials", squash = "Squash", starcraft = "Starcraft", street_fighter_v = "Street Fighter V", sumo = "Sumo", swimming = "Swimming", table_tennis = "Table Tennis", tennis = "Tennis", volleyball = "Volleyball", waterpolo = "Waterpolo", wild_rift = "Wild Rift", winter_sports = "Winter Sports", world_lottery = "World Lottery"
- Sports supported by Cloudbet. See https://gist.github.com/kgravenreuth/6703e1e213aecac4d5728f2f699d34e7#file-sports-json
Side {.pure.} = enum BACK = "BACK", LAY = "LAY"
- Side of the bet.
Procs
proc bet(self: Cloudbet; acceptPriceChange: AcceptPriceChange; currency: CloudbetCurrency; eventId, marketUrl, referenceId: string; price, stake: float; side: Side): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Place a Bet. Bet endpoint has a rate limit of 1 Request per second.
proc getAccountBalance(self: Cloudbet; currency: CloudbetCurrency): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get account balance. Account management handler to show the balance for a requested currency.
proc getAccountCurrencies(self: Cloudbet): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get a list of all currencies available on the account. Account management handler to show the list of currencies currently active on the account.
proc getAccountInfo(self: Cloudbet): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get a all account information. Account management handler to show account specific information.
proc getBetHistory(self: Cloudbet; limit: 1 .. 20 = 20; offset = 0.Natural): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get accepted bet history request with pagination.
proc getBetStatus(self: Cloudbet; referenceId: string): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get bet Status request by Reference ID.
proc getCompetition(self: Cloudbet; competition: string; fromTo: Slice[int]; limit = 50.Positive): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get list of all live and upcoming events of the given competititon key.
proc getCompetition(self: Cloudbet; competition: string; fromTo: Slice[int]; markets: seq[string]; limit = 50.Positive): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get list of all live and upcoming events of the given competititon key.
proc getCompetition(self: Cloudbet; competition: string; limit = 50.Positive): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get list of all live and upcoming events of the given competititon key.
proc getEvent(self: Cloudbet; id: uint): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get 1 Event, shows all available main markets by default.
proc getEvent(self: Cloudbet; id: uint; market: string): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get 1 Event, shows all available main markets by default.
proc getFixtures(self: Cloudbet; sport: CloudbetSport; year: 2000 .. int.high; month: 1 .. 12; day: 1 .. 31; limit = 50.Positive): tuple[ metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get fixtures (i.e. sporting events without markets or metadata) for a given sport on a given date. Shows live and upcoming fixtures of a given sport for a given date. Note that a "day" counts as 00:00 UTC to 23:59 UTC on the requested date.
proc getSport(self: Cloudbet; sport: CloudbetSport): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get 1 Event, shows all available main markets by default.
proc getSports(self: Cloudbet): tuple[metod: HttpMethod, url: Uri, headers: array[3, (string, string)], body: string] {....raises: [], tags: [].}
- Get list of sports in alphabetical order.
func newCloudbet(apiKey: string): Cloudbet {....raises: [], tags: [].}
Macros
macro unrollEncodeQuery(target: var string; args: openArray[(string, auto)]; escape: typed = nil; quote: static[bool] = false)
macro unrollEncodeQuery(target: var string; args: openArray[(string, SomeInteger)]; escape: typed = nil; quote: static[bool] = false)
Templates
template defaultHeaders(self: Cloudbet): array[3, (string, string)]
- Generate default HTTP Headers, the API uses only "application/json" and "X-API-Key" so size is fixed for performance.